When you have a piece of scripting code that you use frequently, it can be better to create a function for this. Instead of copying this piece of code (and having to update each and every instance when you need to change it!), you have it in one central place and can call it whenever you need it.
Creating a function is quite easy. I'll explain based on a simple example: let's say you have a couple of forms that have some sort of Validation workflow, that checks whether a field that needs to be filled in is not empty. If it is empty, you want to show an Alert message, and you want that message to be consistent in those forms. We will put these messages in a Function.
When you are editing an app, take the following steps:
- Go to Workflows --> Functions
- Click "Create Function" or "New Function
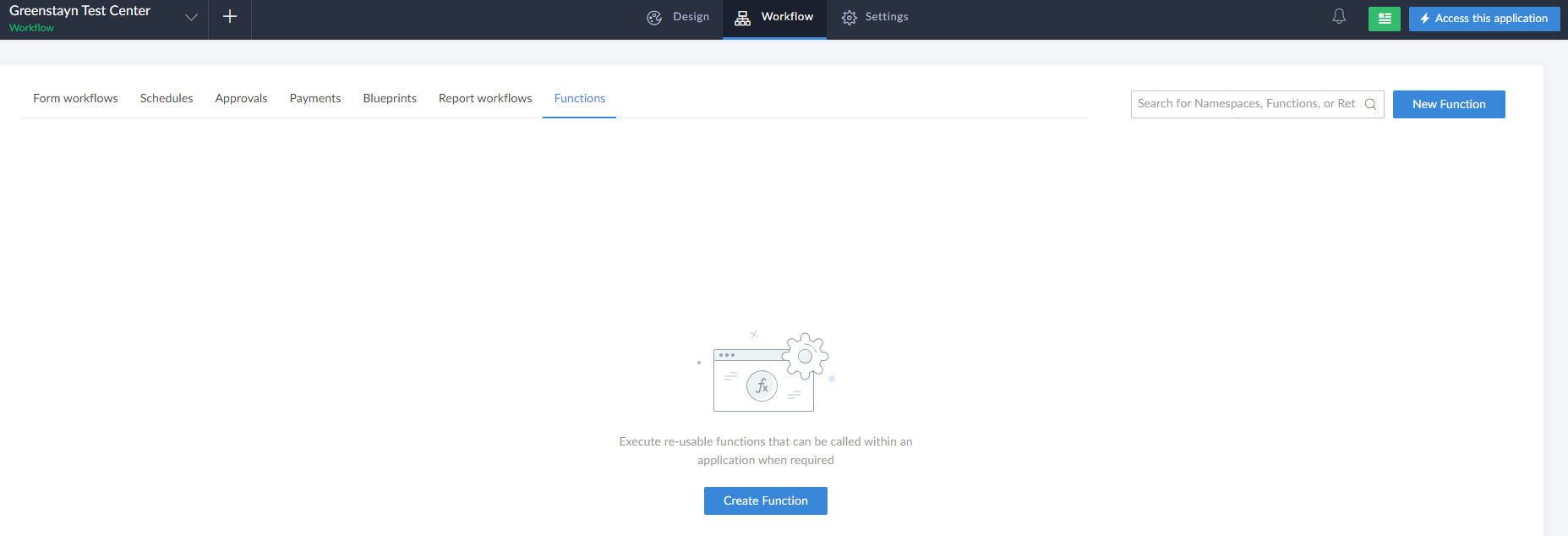
- Fill in the fields. All of these, except for the language, can be changed later.

- Create a name for your function. This is also the name you are going to use when calling the function, so give it something descriptive and easy to recognize. In this case, I called it 'getErrorMessage'.
- Select the language. In this case, we leave it at Deluge, but you can also select Java or NodeJS.
- Select a namespace. You can leave it at Default, but if you're going to add a lot of functions, it is easier to group them in different namespaces. You can always add or change this later. I chose to add a new namespace called 'text'.
- Select the return type. This means the sort of variable you are going to be receiving when calling the function, or "void" if you don't need any feedback. In this case, we want to get a text, so we choose String.
- Then, we create arguments. In this case, I want to be able to get different results, based on which message I want to display. For instance, if I want to display a message for an address field that was not filled in, I want to get a different message than when I want a message for an empty lookup field. I want to say "address" or "lookup" as an argument, which is a string.
- Now that we've created the function, we need to write the script.
You will get an empty function that looks like this:
- string text.getErrorMessage(string msg)
- {
- return "";
- }
The 'return' statement is very important: this is what is going to be sent back when you call the function from your workflow. For now, it's an empty string. It is important that the return is always the very last line in the script. It is also not allowed to have multiple returns. So you can't, for instance, have a list of If and If else statements with a return under every one of those. You should code like this:
- string text.getErrorMessage(string msg)
- {
- text = "";
- if ( msg == "address" )
- {
- text = "Please fill in the address field!";
- }
- else if ( msg == "lookup" )
- {
- text = "Please select a value!" ;
- }
- return text;
- }
Now that we have created the Function, save it. If we want to now call it from a workflow to show the alert message to the user, we can use the following format:
- alert thisapp.text.getErrorMessage("address");
or:
- alert thisapp.text.getErrorMessage("lookup");